사용한 엑셀데이터
1. weapon_data
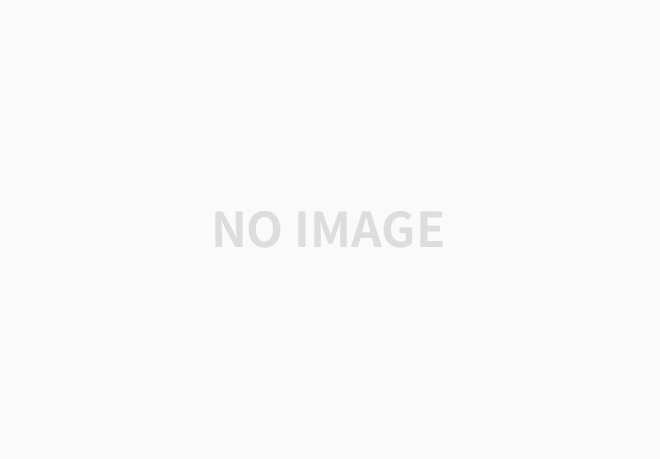
2. effect_data
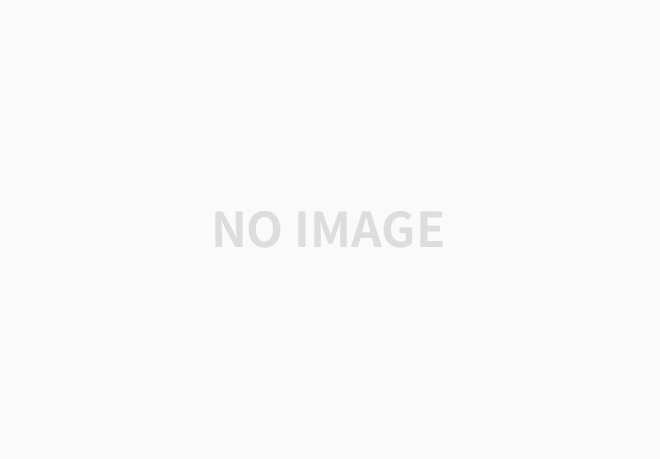
1. UIStudio
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
public class UIStudio : MonoBehaviour
{
public enum eBtnTypes
{
MACHETE,
HAMMER,
AXE
}
public Button[] arrBtns;
public Image weaponIconImage;
public Sprite[] arrSprites;
public Button attackBtn;
private GameObject[] arrWeapons;
private GameObject weaponGo;
private bool isWeapon;
private GameObject[] arrEffects;
private Hero hero;
private Transform dummyRHand;
private List<WeaponData> weaponData;
void Start()
{
this.arrWeapons = new GameObject[3];
this.arrEffects = new GameObject[3];
GameObject heroGo = this.CreateHero();
this.hero.Init(heroGo);
DataManager.GetInstance().Load();
this.weaponData = DataManager.GetInstance().GetWeaponData();
int lastIndex = 0;
for (int i = 0; i < this.arrBtns.Length; i++)
{
int capturedIndx = i;
this.arrBtns[capturedIndx].onClick.AddListener(() =>
{
GameObject.Destroy(this.arrWeapons[lastIndex]);
GameObject.Destroy(this.arrEffects[lastIndex]);
this.weaponIconImage.gameObject.GetComponent<Image>().sprite = this.arrSprites[capturedIndx];
this.CreateWeapon(capturedIndx);
EffectData effectData = DataManager.GetInstance().GetEffectDataById(this.weaponData[capturedIndx].effect_id);
this.EquipWeapon();
this.CreateEffect(capturedIndx, effectData);
lastIndex = capturedIndx;
});
}
this.attackBtn.onClick.AddListener(() =>
{
this.hero.Attack();
});
}
private GameObject CreateHero()
{
GameObject heroShell = new GameObject();
heroShell.name = "Hero";
this.hero = heroShell.AddComponent<Hero>();
GameObject hero = Resources.Load("Prefabs/ch_02_01") as GameObject;
GameObject heroGo = Instantiate(hero) as GameObject;
heroGo.transform.SetParent(heroShell.transform);
this.dummyRHand = heroGo.GetComponent<HeroModel>().dummyRHand;
return heroGo;
}
private void CreateWeapon(int capturedIndx)
{
string weaponPath = string.Format("Prefabs/{0}", this.weaponData[capturedIndx].weapon_res_name);
GameObject weapon = Resources.Load(weaponPath) as GameObject;
this.weaponGo = Instantiate(weapon) as GameObject;
//this.isWeapon = true;
this.weaponGo.transform.localScale = new Vector3(0.2f, 0.2f, 0.2f);
//Debug.Log(weaponGo);
this.arrWeapons[capturedIndx] = weaponGo;
}
private void CreateEffect(int capturedIndx, EffectData effectData)
{
string effectPath = string.Format("Effect/{0}", effectData.effect_res_name);
GameObject effect = Resources.Load(effectPath) as GameObject;
this.arrEffects[capturedIndx] = Instantiate(effect) as GameObject;
this.arrEffects[capturedIndx].transform.position = this.hero.transform.position;
}
private void EquipWeapon()
{
this.weaponGo.transform.SetParent(dummyRHand, false);
this.weaponGo.transform.Rotate(new Vector3(0, 180, 0));
}
void Update()
{
//if (this.isWeapon)
//{
// this.weaponGo.transform.Rotate(100f * Time.deltaTime * Vector3.up);
//}
}
}
|
2. Hero
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Hero : MonoBehaviour
{
private Animation anim;
private string animName;
private float animAttackLength;
private bool isAttack;
private float elapsedTimeCompareAttackEnd;
void Start()
{
Debug.Log("hi");
}
public void Init(GameObject model)
{
this.anim = model.GetComponent<Animation>();
this.animName = "attack_sword_01";
this.animAttackLength = this.anim[animName].length;
}
public void Attack()
{
this.anim.Play(animName);
this.isAttack = true;
}
private void Stop()
{
this.anim.Play("idle@loop");
this.isAttack = false;
}
void Update()
{
if(this.isAttack)
{
this.elapsedTimeCompareAttackEnd += Time.deltaTime;
if(this.elapsedTimeCompareAttackEnd>=this.animAttackLength)
{
this.Stop();
this.elapsedTimeCompareAttackEnd = 0;
}
}
}
}
|
3. HeroModel
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class HeroModel : MonoBehaviour
{
public Transform dummyRHand;
void Start()
{
}
void Update()
{
}
}
|
사용할 Prefab모델에 미리 컴포넌트를 넣고 dummyRHand 오브젝트를 채운뒤 Asset화 한다.
4. DataManager
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
|
using System.Collections;
using System.Collections.Generic;
using System.Runtime.CompilerServices;
using UnityEngine;
using Newtonsoft.Json;
using System.Linq;
public class DataManager
{
private static DataManager Instance;
private Dictionary<int, WeaponData> dicWeaponDatas;
private Dictionary<int, EffectData> dicEffectDatas;
private DataManager()
{
this.dicWeaponDatas = new Dictionary<int, WeaponData>();
this.dicEffectDatas = new Dictionary<int, EffectData>();
}
public static DataManager GetInstance()
{
if(DataManager.Instance==null)
{
DataManager.Instance = new DataManager();
return DataManager.Instance;
}
return DataManager.Instance;
}
public void Load()
{
TextAsset weaponText = Resources.Load("Data/weapon_data") as TextAsset;
string weaponJson = weaponText.text;
TextAsset effectText = Resources.Load("Data/effect_data") as TextAsset;
string effectJson = effectText.text;
this.dicWeaponDatas = JsonConvert.DeserializeObject<WeaponData[]>(weaponJson).ToDictionary(x => x.id, x => x);
this.dicEffectDatas = JsonConvert.DeserializeObject<EffectData[]>(effectJson).ToDictionary(x => x.id, x => x);
}
public List<WeaponData> GetWeaponData()
{
List<WeaponData> weaponDatasList = new List<WeaponData>();
foreach(var pair in this.dicWeaponDatas)
{
weaponDatasList.Add(pair.Value);
}
return weaponDatasList;
}
public EffectData GetEffectDataById(int id)
{
return this.dicEffectDatas[id];
}
}
|
5. WeaponData
1
2
3
4
5
6
7
8
9
10
11
12
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class WeaponData
{
public int id;
public string name;
public string weapon_res_name;
public int effect_id;
}
|
6. EffectData
1
2
3
4
5
6
7
8
9
10
11
12
|
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class EffectData
{
public int id;
public string name;
public string effect_res_name;
}
|
'C# > 수업내용' 카테고리의 다른 글
2020.05.15. 대리자(Delegate) / 이벤트(event) (0) | 2020.05.17 |
---|---|
2020.05.14. 수업내용 - Scene 전환 후 스테이지 클리어하기(ObjectPool) 미완성 (0) | 2020.05.15 |
2020.05.12. 수업내용 - 캐릭터 중심으로 공 공전시키기(Rotate, vector 더하기) (0) | 2020.05.12 |
2020.05.12. 수업내용 - 생성된 캐릭터가 서로 달려와서 공격하기(상속) (0) | 2020.05.12 |
2020.05.11 수업내용 - Scene 변환 (0) | 2020.05.11 |