1. App class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_012
{
class App
{
public App()
{
//캐릭터 데이터 객체 생성
//캐릭터 데이터 멤버: name, hp, damage
//캐릭터 객체 생성시 매개변수로 캐릭터 데이터 객체를 전달
//캐릭터의 이름, 체력, 공격력을 출력
CharacterData data = new CharacterData("홍길동", 100, 2);
Character hong = new Character(data);
Console.WriteLine("이름: {0}, 체력: {1}, 공격력: {2} ", hong.characterData.name, hong.characterData.hp, hong.characterData.damage);
//무기 데이터 객체 생성
WeaponData weaponData = new WeaponData("장검", 10);
//name, damage
//무기 객체를 생성(무기 데이터)
Weapon weapon = new Weapon(weaponData);
//캐릭터 기능
//아이템(무기)을 획득하다
hong.GetWeapon(weapon);
//단검 만들기
WeaponData weaponData1 = new WeaponData("단검", 8);
Weapon weapon1 = new Weapon(weaponData1);
hong.GetWeapon(weapon1);
//소지중인 무기이름 출력하기
hong.PrintWeaponsName();
//홍길동이 갖고 있는 모든 무기들을 가져온다(Weapon객체만 있는 배열을 반환한다)
Weapon[] hongWeapons = hong.GetAllWeapons();
/*
Console.WriteLine("hongWeapons의 길이 : {0}",hongWeapons.Length);
foreach(Weapon allweapon in hongWeapons)
{
if(allweapon!=null)
{
Console.WriteLine(allweapon.weaponData.name);
}
else if(allweapon==null)
{
Console.WriteLine("[ ]");
}
}
*/
//반환받은 Weapon변수에서 문자열 "장검"을 name의 값으로 가지고 있는 waepon객체를 찾으세요
Weapon findWeapon = null;
foreach (Weapon weaponElement in hongWeapons)
{
{
findWeapon = weaponElement;
Console.WriteLine("이름이 장검인 객체: {0}", weaponElement);
}
}
Console.WriteLine();
//찾은 장검을 장비한다.1
//찾은 장검을 장비한다.2
hong.Equip("단검");
Weapon[] arrDropItems = new Weapon[6];
arrDropItems[0] = new Weapon(new WeaponData("창", 11));
arrDropItems[1] = new Weapon(new WeaponData("도끼", 9));
arrDropItems[2] = new Weapon(new WeaponData("활", 6));
arrDropItems[3] = new Weapon(new WeaponData("목검", 5));
arrDropItems[4] = new Weapon(new WeaponData("대검", 8));
arrDropItems[5] = new Weapon(new WeaponData("돌검", 8));
hong.GetWeapon(arrDropItems);
hong.PrintWeaponsName();
}
}
}
|
2. Character class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
221
222
223
224
225
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_012
{
class Character
{
public CharacterData characterData;
public Weapon[] arrWeapons;
public int index;
public Weapon equipWeapon;//착용중인 장비
public Character(CharacterData characterData)
{
this.characterData = characterData;
this.arrWeapons = new Weapon[5];
}
public void GetWeapon(Weapon weapon)
{
this.arrWeapons[this.index] = weapon;
Console.WriteLine("{0}(damage: {1})을(를) 획득하였습니다"
this.index++;
}
public void PrintWeaponsName()
{
Console.WriteLine("\n****소지중인 무기****");
foreach (Weapon weapon in this.arrWeapons)
{
if (weapon != null)
{
Console.WriteLine("이름: {0}({1}), 착용 여부: {2}",weapon.weaponData.name,weapon.weaponData.damage,weapon.isEquiped);
}
}
}
public Weapon[] GetAllWeapons()
{
int length = 0;
foreach (Weapon weapon in this.arrWeapons)
{
if (weapon != null)
{
length++;
}
}
Console.WriteLine("\narrWeapons의 길이 : {0} ", length);
Weapon[] findWeapon = new Weapon[length]; //null이 아닌 값만큼만 길이 만들기
int index = 0;
foreach (Weapon weapon in this.arrWeapons)
{
if (weapon != null)
{
findWeapon[index] = weapon;
index++;
}
}
Console.WriteLine();
return findWeapon;
}
//아이템 장비 1
public void Equip(Weapon weapon)
{
this.equipWeapon = weapon;
this.equipWeapon.isEquiped = true; //아이템 착용 중으로 변경
}
//아이템 장비 2
public void Equip(string name)
{
Weapon foundWeapon =null;
if (this.equipWeapon != null)
{
this.equipWeapon.isEquiped = false;
}
foreach (Weapon weapon in this.arrWeapons)
{
if (weapon != null)
{
{
foundWeapon = weapon;
break;
}
}
}
//만약에 아이템을 찾았다면
if(foundWeapon!=null)
{
if(this.equipWeapon!=null)
{
this.equipWeapon.isEquiped = false;
}
this.equipWeapon = foundWeapon;
this.equipWeapon.isEquiped = true;
}
//아이템을 출력한다
/*foreach(Weapon weapon in this.arrWeapons)
{
if(weapon!=null)
{
Console.WriteLine("{0} {1}",weapon.weaponData.name,this.equipWeapon.isEquiped);
}
}*/
}
public void GetWeapon(Weapon[] weapons)
{
//기존 배열의 길이
int myArrLength = 0;
Weapon[] newArrweapon = null;
int newIndex = 0;
foreach (Weapon weapon in this.arrWeapons)
{
if (weapon != null)
{
myArrLength++;
}
}
Console.WriteLine("사용중인 공간: {0}", myArrLength);
{
newArrweapon = new Weapon[newLength];
//기존값부터 넣기
{
if(this.arrWeapons[i]!=null)
{
newArrweapon[newIndex] = this.arrWeapons[i];
newIndex++;
}
}
Console.WriteLine(newIndex);
{
if(weapons!=null)
{
newArrweapon[newIndex] = weapons[j];
newIndex++;
}
}
Console.WriteLine(newIndex);
}
//길이가 충분할때
{
{
if(weapons!=null)
this.arrWeapons[this.index] = weapons[i];
index++;
}
}
//새로운 배열 출력하기
foreach(Weapon weapon in newArrweapon)
{
if(weapon!=null)
{
Console.WriteLine("{0}({1}), 착용여부: {2}",weapon.weaponData.name,weapon.weaponData.damage,weapon.isEquiped);
}
}
//새 배열을 기존 배열에 넣기
this.arrWeapons = newArrweapon;
}
}
}
|
3. CharacterData class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_012
{
class CharacterData
{
public string name;
public int hp;
public int damage;
public CharacterData(string name, int hp, int damage)
{
this.name = name;
this.hp = hp;
this.damage = damage;
}
}
}
|
4. Weapon class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_012
{
class Weapon
{
public WeaponData weaponData;
public bool isEquiped; //착용중인지 아닌지
public Weapon(WeaponData weaponData)
{
this.weaponData = weaponData;
this.isEquiped = false;
}
}
}
|
5. WeaponData class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_012
{
class WeaponData
{
public string name;
public int damage;
public WeaponData(string name, int damage)
{
this.name = name;
this.damage = damage;
}
}
}
|
6. 결과값
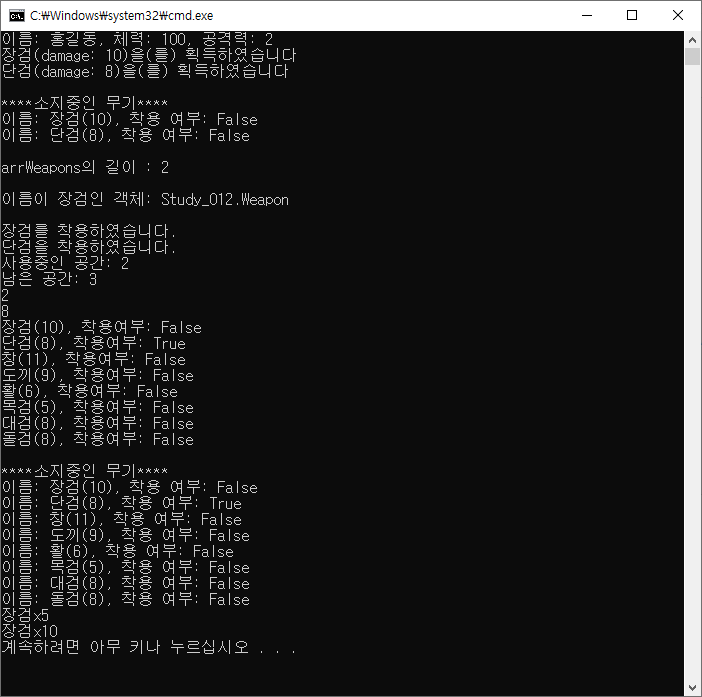
'C# > 수업내용' 카테고리의 다른 글
2020.04.21. 수업내용 - Dictionary 개요 (0) | 2020.04.21 |
---|---|
2020.04.20. 수업내용 - 아이템 수량만 늘리기(객체 생성 x) (0) | 2020.04.21 |
2020.04.16. 수업내용 - 1) 문자열 분할하고 배열객체에 할당하기, 2) 배열 객체를 다른 클래스에 전달하기 (0) | 2020.04.16 |
2020.04.16. 수업내용 - 장바구니 (장바구니 클래스, 과일 클래스) (0) | 2020.04.16 |
2020.04.16. 수업내용 - 문자열 배열 출력, 배열값 거꾸로 출력하기(foreach) (0) | 2020.04.16 |