1. App class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_010
{
class App
{
public App()
{
inventory inventory = new inventory();
//문자열 분할하기
string itemName ="장검,단검,활";
//문자열 배열길이와 똑같은 길이를 가진 아이템 배열 생성하기
//반복문으로 아이템 객체만들고 아이템 배열객체에 할당하기
{
arritems[i]=new Item(strItemName[i]);
}
//Inventory 클래스 AddItem 메서드에 배열인스턴스를 값으로 전달하기
inventory.AddItem(arritems);
//출력하기
inventory.PrintItemLise();
}
}
}
|
2. Inventory class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_010
{
class inventory
{
Item[] arritems;
public inventory()
{
this.arritems = new Item[5];
}
//App에서 만든 배열객체를 inventory 클래스에 배열 옮겨담기
public void AddItem(Item[] arritems)
{
Console.WriteLine("배열객체 추가하기\n");
{
this.arritems[i] = arritems[i];
Console.WriteLine("인덱스 {0} : {1} 추가 ",i,this.arritems[i].GetName());
}
}
public void PrintItemLise()
{
Console.WriteLine("\n목록 출력하기\n");
{
if(this.arritems[i]==null)
{
return;
}
else
{
Console.WriteLine("인덱스 {0} 의 값 : {1}", i, this.arritems[i].GetName());
}
}
}
}
}
|
3. Item class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_010
{
class Item
{
string itemName;
public Item(string itemName)
{
this.itemName = itemName;
}
//이름 알려주기
public string GetName()
{
return this.itemName;
}
}
}
|
4. 결과값
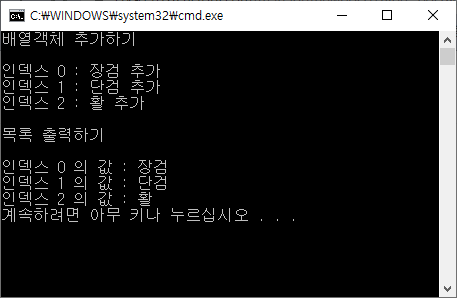
'C# > 수업내용' 카테고리의 다른 글
2020.04.20. 수업내용 - 아이템 수량만 늘리기(객체 생성 x) (0) | 2020.04.21 |
---|---|
2020.04.20. 수업내용 - data 클래스 따로 만들기 (0) | 2020.04.21 |
2020.04.16. 수업내용 - 장바구니 (장바구니 클래스, 과일 클래스) (0) | 2020.04.16 |
2020.04.16. 수업내용 - 문자열 배열 출력, 배열값 거꾸로 출력하기(foreach) (0) | 2020.04.16 |
2020.04.14. 수업내용 - Barracks, Unit, Academy 클래스 (0) | 2020.04.14 |