사용할 엑셀 데이터
1. App class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
|
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Text;
using Newtonsoft.Json;
namespace Study_014_1
{
class App
{
public Dictionary<int, achievementData> dicAchievementDatas;
Dictionary<int, achievementInfo> dicAchievementInfos;
public achievementData[] arrAchievementDatas;
public App()
{
string json = File.ReadAllText(path);
arrAchievementDatas = JsonConvert.DeserializeObject<achievementData[]>(json);
dicAchievementDatas = new Dictionary<int, achievementData>();
foreach (achievementData data in arrAchievementDatas)
{
}
//Info를 저장할 Dictionary
dicAchievementInfos = new Dictionary<int, achievementInfo>();
//기존 유저인지
{
string infojson = File.ReadAllText(infopath);
achievementInfo[] arrAchievementinfos = JsonConvert.DeserializeObject<achievementInfo[]>(infojson);
foreach (achievementInfo data in arrAchievementinfos)
{
}
}
//신규 유저인지
else
{
//info에 data를 저장한다.
foreach (KeyValuePair<int, achievementData> pair in dicAchievementDatas)
{
achievementData data = pair.Value;
}
this.SaveData();
}
//업적 보기
this.PrintAchievement(1);
this.DoAchieve(1);
this.SaveData();
}
public void PrintAchievement(int id)
{
achievementData data = dicAchievementDatas[id];
achievementInfo info = dicAchievementInfos[id];
foreach (KeyValuePair<int, achievementData> pairs in dicAchievementDatas)
{
{
Console.WriteLine("id: {0},\t\t이름: {1},\t목표: ({2}/{3})", pairs.Key, data.name, info.count, data.goal);
Console.WriteLine("보상: {0},\t완료여부: {1},\t설명: {2}", data.reward_amount, data.achievement, data.desc);
}
}
}
public void DoAchieve(int id)
{
achievementData data = dicAchievementDatas[id];
achievementInfo info = dicAchievementInfos[id];
Console.WriteLine("\n업적을 1개 완료하였습니다\n");
foreach (KeyValuePair<int, achievementData> pairs in dicAchievementDatas)
{
{
Console.WriteLine("id: {0},\t\t이름: {1},\t목표: ({2}/{3})", pairs.Key, data.name, info.count, data.goal);
Console.WriteLine("보상: {0},\t완료여부: {1},\t설명: {2}", data.reward_amount, data.achievement, data.desc);
}
}
}
public void SaveData()
{
achievementInfo[] newArr = new achievementInfo[length];
int index = 0;
foreach(KeyValuePair<int, achievementInfo> pair in dicAchievementInfos)
{
newArr[index] = pair.Value;
index++;
}
string jsonInfo = JsonConvert.SerializeObject(newArr);
File.WriteAllText(path, jsonInfo);
}
}
}
|
2. achievementData class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_014_1
{
class achievementData
{
public int id;
public string name;
public int goal;
public int reward_amount;
public bool achievement;
public string desc;
}
}
|
3. achievementInfo class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_014_1
{
class achievementInfo
{
public int id;
public int count;
public achievementInfo(int id, int count)
{
this.id = id;
this.count = count;
}
}
}
|
4. 결과값
1)처음 실행하고 업적을 완료하고 저장했을 때
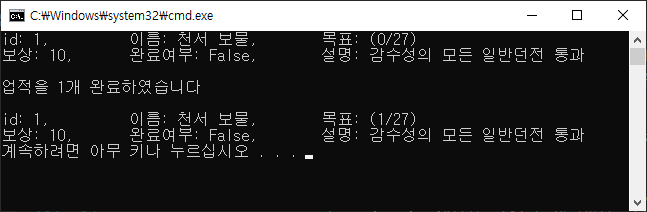
2) 다시 실행하고 업적을 출력만 했을때 (저장파일 있을때)

3) 저장파일을 없애고 다시 실행하여 출력만 했을 때 (저장파일 없을 때)

'C# > 수업내용' 카테고리의 다른 글
2020.04.23. 수업내용 - json 파일 읽고 쓰기(불러오기, 저장하기) 3, 파일 2개 연습 (0) | 2020.04.23 |
---|---|
2020.04.23. 수업내용 - json 파일 읽고 쓰기(불러오기, 저장하기) 2 (0) | 2020.04.23 |
2020.04.21. 수업내용 - Json 파일 만들고 프로그램에 넣기 (0) | 2020.04.21 |
2020.04.21. 수업내용 - Dictionary 개요 (0) | 2020.04.21 |
2020.04.20. 수업내용 - 아이템 수량만 늘리기(객체 생성 x) (0) | 2020.04.21 |