1. App class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_009
{
class App
{
public App()
{
//인벤토리 생성
Inventory inventory = new Inventory();
//아이템 생성 및 배열에 추가하는 메서드 호출
Item item = new Item("장검");
inventory.AddItem(item);
inventory.AddItem(new Item("단검"));
inventory.AddItem(new Item("활"));
inventory.AddItem(new Item("도끼"));
inventory.AddItem(new Item("창"));
//아이템 이름 출력 메서드 호출
Console.WriteLine("\n[배열 출력하기]");
inventory.PrintItemNames();
//아이템 찾기 메서드 호출
Console.WriteLine("\n[아이템 찾기]");
Item foundItem = inventory.FindItem("단검");
//아이템 빼기 메서드 호출
Console.WriteLine("\n[아이템 빼기]");
Item getItem=inventory.GetItem("단검");
//출력 메서드 호출
Console.WriteLine("\n[배열 출력하기]");
inventory.PrintItemNames();
//null값을 가장 마지막 배열인덱스에 넣는 정리 메서드 호출
Console.WriteLine("\n[배열 정리하기]");
inventory.arrangeIndex();
//출력 메서드 호출
Console.WriteLine("\n[배열 출력하기]");
inventory.PrintItemNames();
}
}
}
|
2. Inventory class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_009
{
class Inventory
{
public Item[] items;
int index;
public Inventory()
{
this.items = new Item[5];
Console.WriteLine("인벤토리가 생성되었습니다.\n");
}
//아이템 추가하기
public void AddItem(Item item)
{
this.items[this.index] = item;
this.index++;
}
//아이템 이름 출력하기
public void PrintItemNames()
{
Console.WriteLine();
{
if (this.items[i] == null)
{
Console.WriteLine("배열 인덱스 {0}의 값 : [ ]", i);
}
else
{
Console.WriteLine("배열 인덱스 {0}의 값 : {1}", i, this.items[i].itemName);
}
}
}
//아이템 찾기
public Item FindItem(string itemName)
{
Console.WriteLine();
Item foundItem = null; //찾은 값을 저장할 변수
foreach (Item item in this.items)
{
if (item != null)
{
Console.WriteLine("찾는 값: {0}, 비교하는 값: {1}", itemName, item.itemName);
if (item.itemName == itemName)
{
Console.WriteLine("{0}을(를) 찾았습니다.", itemName);
foundItem = item;
break;
}
}
}
return foundItem;
}
//아이템 빼서 null로 바꿔주기
//전달된 아이템을 먼저 찾고
//뺀 값은 반환하고
//그 자리에 null을 넣어준다.
public Item GetItem(string itemName)
{
Console.WriteLine();
Console.WriteLine("{0}을(를) 찾습니다", itemName);
Item getItem = null;
{
if (this.items[i] != null)
{
if (this.items[i].itemName == itemName)
{
Console.WriteLine("{0}을(를) 반환하고 해당 인덱스 값을 비웁니다.", itemName);
getItem = this.items[i];
this.items[i] = null;
break;
}
}
}
return getItem;
}
//배열 인덱스 비어있는 값 정리하기
//배열 인덱스 값이 null인지 비교한다
//null이면 비교되는 해당 인덱스(i)에 바로 뒤(i+1)의 배열값을 넣는다.
//바로 뒤의 값(i+1)에 null을 넣는다
//null이 가장 끝자리(length-1)가 될때까지 반복한다.
public void arrangeIndex()
{
Console.WriteLine("\n배열을 정리합니다.");
int i = 0;
while (true)
{
if (this.items[i] == null)
{
this.items[i] = this.items[i + 1];
this.items[i + 1] = null;
}
i++;
{
break;
}
}
}
}
}
|
3. Item class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_009
{
class Item
{
public string itemName;
public Item(string itemName)
{
this.itemName = itemName;
Console.WriteLine("{0}이(가) 생성되었습니다.",this.itemName);
}
}
}
|
4. 결과 값
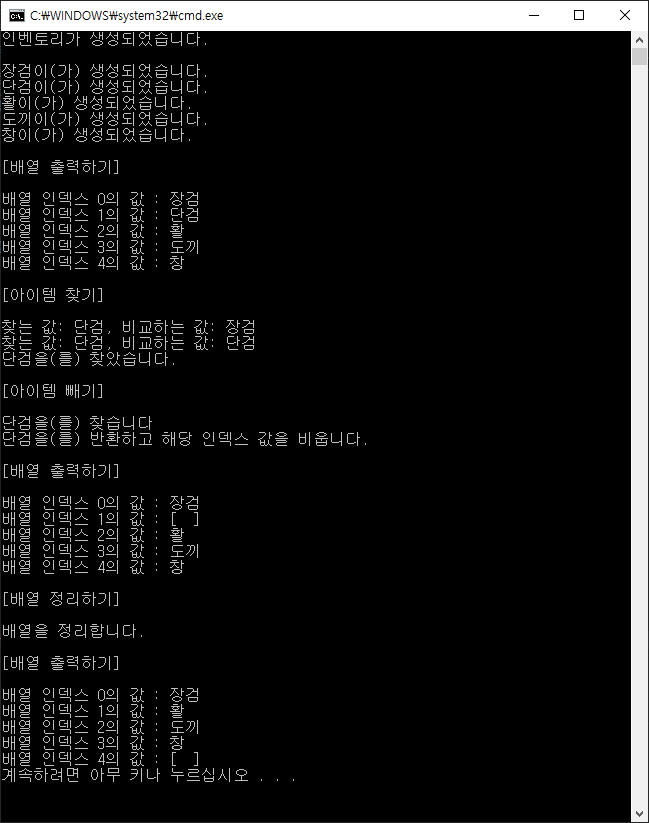
'C# > 과제' 카테고리의 다른 글
2020.04.20. 과제 - 길바닥 아이템 획득하기(컬렉션(list)) (0) | 2020.04.21 |
---|---|
2020.04.18. 과제 - 음식 만들기 (배열(정렬), 클래스) (0) | 2020.04.18 |
2020.04.10. 과제 - Character class, Item class (0) | 2020.04.10 |
2020.04.08. 종족, 직업 선택하기 (switch, 열거형 변환) (0) | 2020.04.08 |
2020.04.03. 과제 - 이중 for문 (0) | 2020.04.03 |