1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
//14. 문자열 나누기 1 - substring
string str = "1234회";
string SubStr = str.Substring(2); //문자열을 나눈다.
Console.WriteLine(SubStr);
Console.WriteLine("--------------------------------------------");
//15. 문자열 나누기 2 - split
string text2 = "12회34회56회";
char sp = '회'; //'회'를 기준으로 문자열을 나눌거임
Console.WriteLine(text2);
string[] spstring = text2.Split(sp); //sp (회)를 기준으로 문자열을 나눠서 spstring값에 넣는다.
Console.WriteLine(spstring.Length); //spstring의 길이를 출력한다.
Console.WriteLine(spstring[2]); //spstring에 저장된 값중에 3번째에 뭐가 있는지 출력해보자.
Console.WriteLine("--------------------------------------------");
//16. split 응용하기 - 'n회'를 입력해도 n만을 입력받아 버그없이 for문이 작동한다.
Console.Write("몬스터를 몇번 공격하겠습니까? ");
string strCount1=Console.ReadLine();
char c = '회';
string[] strCount2=strCount1.Split(c);
int count=Convert.ToInt32(strCount2[0]);
for(int i=1; i<=count; i++)
{
Console.WriteLine("몬스터를 "+i+"번 공격했습니다!");
}
|
★ Console.WriteLine(spstring.Length); 의 값이 4가 나오는 이유
마지막 '회' (56회) 뒤에 null 값이 있기 때문이다.
1
2
3
4
5
6
7
8
9
10
|
string str2 = "12회34회56회";
char s = '회';
Console.WriteLine("--> " + spstring2[3]);
Console.WriteLine("--> " + spstring2[3].GetType());
Console.WriteLine("--> " + (spstring2[3] == String.Empty));
|
1) GetType();
현재 인스턴스의 Type을 알려준다 (반환한다).
2) String.Empty
boolean 형식으로
지정된 문자열이 null이거나 비어있으면 true 값
뭐가 있으면 false 값을 나타낸다.
비교) 뭐가 있을 때
1
2
3
4
5
6
7
8
|
string str2 = "12회34회56회";
char s = '회';
Console.WriteLine("--> " + spstring2[2]);
Console.WriteLine("--> " + spstring2[2].GetType());
Console.WriteLine("--> " + (spstring2[2] == String.Empty));
|
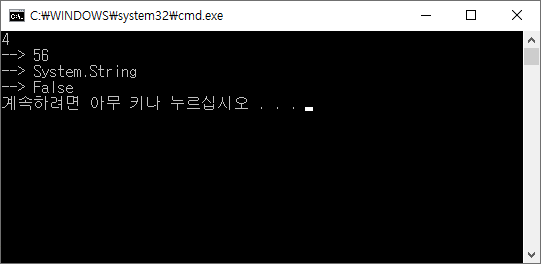
참고 :
1) Object.GetType 메서드, MS Docs
https://docs.microsoft.com/ko-kr/dotnet/api/system.object.gettype?view=netframework-4.8
2) String.IsNullOrEmpty(String)메서드, Ms Docs
https://docs.microsoft.com/ko-kr/dotnet/api/system.string.isnullorempty?view=netframework-4.8
'C# > 수업내용' 카테고리의 다른 글
2020.04.06. 수업내용 - for문, if문 1 (목록, 쉼표 빼기) (0) | 2020.04.06 |
---|---|
2020.04.06. 수업내용 - 문자열 표현식, var변수 타입 (0) | 2020.04.06 |
2020.04.03. 수업내용 - break 문, continue 문 (0) | 2020.04.04 |
2020.04.03. 수업내용 - ReadLine, Convert.ToInt32, Int32.Parse (0) | 2020.04.04 |
2020.04.03. 수업내용 - for문, 글자색 변경 (0) | 2020.04.03 |