엑셀테이블
1) 재화 테이블(다이아몬드, 골드)
2) 상점 항목 테이블
3) 시간 테이블
1. App class
재접속 시 시간이 하루이상(double target=24) 차이가 날때 유저의 시간 값을 현재 날짜의 자정으로 바꿨습니다.
daily 상품이 아닌 경우 UserInfo 객체의 리스트에 저장된 해당 Id의 갯수를 세어 구매한 카운터로 사용했습니다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
122
123
124
125
126
127
128
129
130
131
132
133
134
135
136
137
138
139
140
141
142
143
144
145
146
147
148
149
150
151
152
153
154
155
156
157
158
159
160
161
162
163
164
165
166
167
168
169
170
171
172
173
174
175
176
177
178
179
180
181
182
183
184
185
186
187
188
189
190
191
192
193
194
195
196
197
198
199
200
201
202
203
204
205
206
207
208
209
210
211
212
213
214
215
216
217
218
219
220
|
using Newtonsoft.Json;
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime;
using System.Security.Cryptography.X509Certificates;
using System.Text;
using System.Threading;
namespace Study_018
{
class App
{
public UserInfo userInfo;
public App()
{
DataManager dataManager = DataManager.GetInstance();
dataManager.LoadData();
if (File.Exists(path))
{
Console.WriteLine("기존 유저");
string saveJson = File.ReadAllText(path);
userInfo = JsonConvert.DeserializeObject<UserInfo>(saveJson);
DateTime usertime = userInfo.time;
DateTime now = DateTime.Now;
TimeSpan result = now - usertime;
double target = 24;
if(result.TotalHours>target)
{
Console.WriteLine("날짜 변경");
userInfo.dailycount = 0;
}
}
else
{
userInfo = new UserInfo();
//테스트
Console.WriteLine("신규 유저");
this.SaveData();
}
this.PrintProduct(1005);
this.Purchase(1005);
this.PrintProduct(1005);
this.SaveData();
}
public void PrintProduct(int id)
{
Console.WriteLine("-----------------------------------------------------");
ProductData product = DataManager.GetInstance().GetProductDataById(id);
BudgetData budget = DataManager.GetInstance().GetBudgetDataByType(product.budget_type);
TimeData timedata = DataManager.GetInstance().GetTimeDataByType(product.time_type);
if(product.str_mileage!="null")
{
Console.WriteLine("{0}{1}",product.str_mileage,product.mileage_amount);
}
if(product.require_level!=0)
{
Console.WriteLine("레벨 {0}이상", product.require_level);
}
Console.WriteLine("icon: "+product.icon_name);
if(product.is_daily)
{
Console.WriteLine("매일 {0}/{1}", userInfo.dailycount, product.amount_limit);
Console.WriteLine("매일매일 추천!");
}
else
{
int count = 0;
foreach (var data in userInfo.productInfosList)
{
{
count++;
}
}
if(product.amount_limit != 0 || count == 0 || count < product.amount_limit)
{
Console.WriteLine("({0}/{1})",count,product.amount_limit);
}
}
if(product.time_type==1)
{
return;
}
else
{
DateTime time = Convert.ToDateTime(timedata.time);
DateTime now = DateTime.Now;
TimeSpan result = time - now;
Console.WriteLine("{0} {1}일 {2}시간 {3}분", timedata.str_time, result.Days, result.Hours, result.Minutes);
}
}
public void Purchase(int id)
{
Console.WriteLine("-----------------------------------------------------");
ProductData product = DataManager.GetInstance().GetProductDataById(id);
{
if(product.is_daily)
{
if(userInfo.dailycount<product.amount_limit)
{
{
userInfo.dailycount++;
return;
}
else
{
return;
}
}
else
{
Console.WriteLine("구매할 수 없습니다.");
return;
}
}
else
{
int count = 0;
foreach (var data in userInfo.productInfosList)
{
{
count++;
}
}
if (product.amount_limit==0||count == 0||count < product.amount_limit)
{
return;
}
else
{
Console.WriteLine("더이상 구매할 수 없습니다.");
}
}
}
else
{
Console.WriteLine("레벨이 낮아 구매할 수 없습니다.");
return;
}
}
public void SaveData()
{
//직렬화 하고
string saveJson = JsonConvert.SerializeObject(userInfo);
//저장하기
File.WriteAllText("./data/save_data.json", saveJson);
}
}
}
|
2. DataManager class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
|
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Runtime.CompilerServices;
using System.Runtime.Remoting.Metadata.W3cXsd2001;
using System.Text;
using Newtonsoft.Json;
namespace Study_018
{
class DataManager
{
private static DataManager instance;
Dictionary<int, ProductData> dicProductDatas;
Dictionary<int, BudgetData> dicBudgetDatas;
Dictionary<int, TimeData> dicTimeDatas;
private DataManager()
{
this.dicProductDatas = new Dictionary<int, ProductData>();
this.dicBudgetDatas = new Dictionary<int, BudgetData>();
this.dicTimeDatas = new Dictionary<int, TimeData>();
}
public static DataManager GetInstance()
{
if(DataManager.instance==null)
{
DataManager.instance = new DataManager();
return DataManager.instance;
}
return DataManager.instance;
}
public void LoadData()
{
this.dicProductDatas = JsonConvert.DeserializeObject<ProductData[]>(productJson).ToDictionary(x => x.id, x => x);
this.dicBudgetDatas = JsonConvert.DeserializeObject<BudgetData[]>(budgetJson).ToDictionary(x => x.id, x => x);
this.dicTimeDatas = JsonConvert.DeserializeObject<TimeData[]>(timeJson).ToDictionary(x => x.id, x => x);
}
public ProductData GetProductDataById(int id)
{
return this.dicProductDatas[id];
}
public BudgetData GetBudgetDataByType(int type)
{
BudgetData data = null;
foreach(var pair in this.dicBudgetDatas)
{
{
data = pair.Value;
}
}
return data;
}
public TimeData GetTimeDataByType(int type)
{
TimeData data = null;
foreach(var pair in this.dicTimeDatas)
{
{
data = pair.Value;
}
}
return data;
}
}
}
|
3. UserInfo class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_018
{
class UserInfo
{
public int level=1;
public DateTime time;
public List<ProductInfo> productInfosList;
public int dailycount=0;
public UserInfo()
{
productInfosList = new List<ProductInfo>();
}
}
}
|
4. ProductInfo class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
|
using System;
using System.Collections.Generic;
using System.Data.SqlTypes;
using System.Linq;
using System.Text;
namespace Study_018
{
class ProductInfo
{
public int id;
public int amount;
public ProductInfo(int id, int amount)
{
this.id = id;
this.amount = amount;
}
}
}
|
5. BudgetData class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_018
{
class BudgetData
{
public int id;
public int budget_type;
public string name;
public string icon_name;
}
}
|
6. ProductData class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_018
{
class ProductData
{
public int id;
public string name;
public int amount;
public int amount_limit;
public string str_mileage;
public int mileage_amount;
public int require_level;
public int budget_type;
public int price;
public bool is_daily;
public int time_type;
public string icon_name;
}
}
|
7. TimeData class
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
namespace Study_018
{
class TimeData
{
public int id;
public int time_type;
public string str_time;
public string time;
}
}
|
8. 결과값
1)
처음으로 실행했을때(저장 파일이 없을 때)
마지막 실행시간(2019-01-01, 신규 유저일 때 초기화)
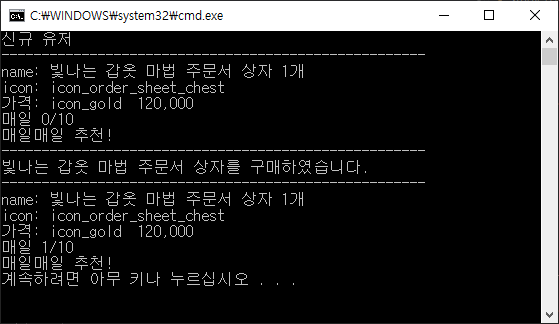
2)
다시 실행할 경우 유저의 마지막 실행시간을 현재 날짜의 자정으로 변경
2019-01-01 -> 2020.04.29. 12:00:00
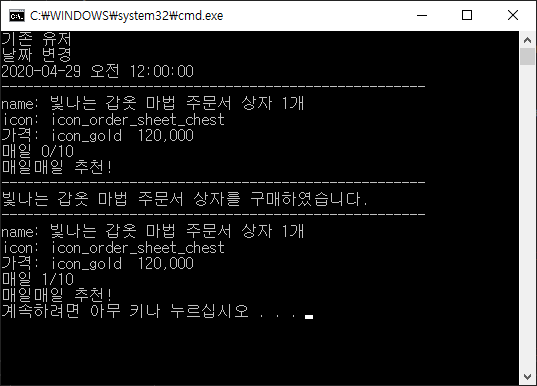
3) 데일리 상품이 아닌 다른 상품을 구매했을 때
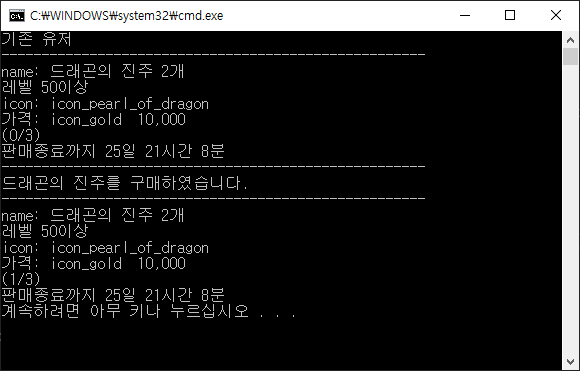
4) 구매제한 횟수를 넘었을 때
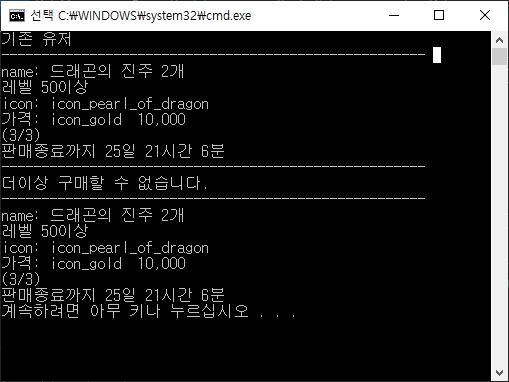
'C# > 수업내용' 카테고리의 다른 글
2020.04.29. 수업내용 - 일일 출석 보상(+연속 출석 보상) (0) | 2020.04.30 |
---|---|
2020.04.29. 수업내용 - 스킨목록 출력하기 (0) | 2020.04.29 |
2020.04.26. 승급하기 연습 (메이플 스토리) (0) | 2020.04.26 |
2020.04.24. 수업내용 - 승급하기(바람의 나라) (0) | 2020.04.25 |
2020.04.23. 수업내용 - 시간 지나면 하트주기(DateTime) (0) | 2020.04.24 |