JEST는 Test Runner, Test Matcher, Test Mock 를 제공해주는 테스트 프레임워크이다.
설치
npm install --save-dev jest
설치 후엔 package.json 을 열어서 script 의 test 부분을 지우고 jest 로 고쳐주면
npm test로 테스트 할 수 있다.
"scripts": {
"test": "jest"
},
테스트 파일을 만들 땐, 테스트 파일명.test.js로 만든다. (app.test.js)
npm test를 실행하면 Jest는 기본적으로 test.js로 끝나거나, __test__ 디렉터리 안에 있는 파일들을 테스트 파일로 인식하여
프로젝트 내에 모든 테스트 파일을 찾아서 테스트를 실행한다.
만약 특정 테스트 파일만 실행하고 싶은 경우에는 npm test 파일명 또는 경로를 입력한다.
1. Matcher
expect(a).toXXXXX(3); 이런 구문에서 expect안의 값은 검증대상, 3은 기대값이다.
toXXXXXX 부분은 Test Matcher 라고 한다.
fn.js
const fn = {
add: (a, b) => a + b,
}
module.exports = fn;
fn.test.js
const fn = require("./fn");
//describe는 테스트를 그룹으로 묶어준다.
describe("matcher", () => {
//pass
test("1 === 1", () => {
//toBe부분은 Matcher라고 한다.
expect(1).toBe(1);
});
//pass
test("2 + 3 === 5", () => {
expect(fn.add(2, 3)).toBe(5);
});
//fail
test("3 + 3 === 5", () => {
expect(fn.add(3, 3)).toBe(5);
});
//pass
test("3 + 3 !== 5", () => {
expect(fn.add(3, 3)).not.toBe(5);
});
//pass
test("2 + 3 === 5", () => {
expect(fn.add(2, 3)).toEqual(5);
});
});
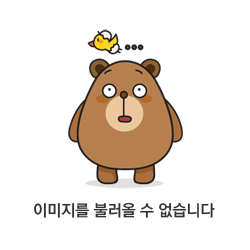
1.1. toBe 와 toEqual
toBe는 값이 같은지, 객체도 같은지 비교한다.(===와 비슷)
toEqual은 값이 같은 지 비교한다.
추가적으로 toStrictEqual 함수는 엄격하게 확인하는 데 값에 undefined가 포함되어 있으면 실패한다.
fn.js 에 유저 객체를 반환하는 함수를 추가해준다.
makeUser: (name, age) => ({ name, age, gender: undefined }),
fn.test.js에 테스트를 추가해주고 테스트를 실행한다.
//fail
//toBe - 객체가 같아보여도 다른 메모리에 있는 객체이기 때문에 fail
test("toBe를 사용해 이름과 나이를 받아서 객체를 반환", () => {
expect(fn.makeUser("Kim", 30)).toBe({
name: "Kim",
age: 30,
});
});
//pass
//toEqual - 객체의 내용이 같으면 pass
test("to equal를 사용해 이름과 나이를 받아서 객체를 반환", () => {
expect(fn.makeUser("Kim", 30)).toEqual({
name: "Kim",
age: 30,
});
});
//fail
//toStrictEqual - undefined 허용 안함(gender: undefined)
test("toStrictEqual를 사용해 이름과 나이를 받아서 객체를 반환", () => {
expect(fn.makeUser("Kim", 30)).toStrictEqual({
name: "Kim",
age: 30,
});
});
1.2. toBeCloseTo
공식문서에는 toBe로 소수점을 확인하지 말라고 써있는데 자바스크립트에서는 소수점을 계산했을 때 딱 떨어지지 않기 때문이다.
ex) 0.1 + 0.2 = 0.30000000000000004
대신에 toBeCloseTo를 사용해 근사값을 구해 비교한다.
Don't use .toBe with floating-point numbers. For example, due to rounding, in JavaScript 0.2 + 0.1 is not strictly equal to 0.3. If you have floating point numbers, try .toBeCloseTo instead.
.toBeCloseTo(number, numDigits?)
옵션인 numDigits은 확인할 소수점 자리 수를 제한하는데 기본값은 2이다.
//toBeCloseTo - 근사치 구하기
test("0.1 + 0.2 = 0.3", () => {
//fail
expect(fn.add(0.1, 0.2)).toBe(0.3);
//pass
expect(fn.add(0.1, 0.2)).toBeCloseTo(0.3);
});
1.3. null, true, false 체크
toBeNull은 null 값인지 확인한다.
toBeTruthy는 검증값이 true인지,
toBeFalsy는 검증값이 false인지 확인한다.
//null check - pass
test("is null", () => {
expect(null).toBeNull();
});
//true check - pass
test("is true?", () => {
expect(fn.add(1, 1)).toBeTruthy();
});
//false check - pass
test("is false?", () => {
expect(fn.add(1, -1)).toBeFalsy();
});
1.4. 숫자 값 비교
toBeGreaterThan 기대값을 초과하는 지
toBeGreaterThanOrEqual 기대값과 같거나 큰지
toBeLessThan 기대값 미만인지
toBeLessThanOrEqual 기대값과 같거나 작은지
fn.js 에 문자열 길이를 반환하는 함수 추가
returnTextLength: (string) => string.length,
//toBeGreaterThan - pass
test("n를 초과함?", () => {
expect(fn.returnTextLength("abcde")).toBeGreaterThan(4);
});
//toBeGreaterThanOrEqual - pass
test("n과 같거나 큼?", () => {
expect(fn.returnTextLength("abcde")).toBeGreaterThanOrEqual(5);
});
//toBeLessThan - pass
test("n 미만임?", () => {
expect(fn.returnTextLength("abcde")).toBeLessThan(6);
});
//toBeLessThanOrEqual - pass
test("n과 같거나 작음?", () => {
expect(fn.returnTextLength("abcde")).toBeLessThanOrEqual(5);
});
1.5. 정규식과 매치
toMatch는 문자열이 정규식과 매치되는 지 확인할 때 사용
//정규식과 매치
test("abcde에서 a가 있나?", () => {
//pass
expect("abcde").toMatch(/a/);
//pass
expect("abcde").toMatch(/A/i);
//fail
expect("abcde").toMatch(/f/);
});
1.6. 배열에 값 포함 여부
toContain은 배열에 값이 포함되어있는 지 확인
//toContain
test("배열에 a가 있나?", () => {
//pass
expect(["a", "b", "c"]).toContain("a");
//fail
expect(["a", "b", "c"]).toContain("d");
});
1.7. 예외처리(에러) 확인
toThrow 예외처리(에러)를 확인할 수 있음
인자를 넘겨주면 예외 메시지를 비교할 수 있음(정규식도 가능)
fn.js 에 에러를 발생시키는 함수 추가
throwErr: () => {
throw new Error("1번 에러");
},
//toThrow 예외처리가 발생했는지?
test("1번 에러남?", () => {
//pass
expect(() => fn.throwErr()).toThrow();
//pass
expect(() => fn.throwErr()).toThrow("1번 에러");
//fail
expect(() => fn.throwErr()).toThrow("2번 에러");
});
1.8. 비동기 처리 테스트
fn.js에 3초 후에 callback을 실행하는 함수와 promise 를 반환하는 함수 추가
callbackTest: (callback) => {
const name = "Kim";
setTimeout(() => {
callback(name);
// throw new Error("서버 에러");
}, 3000);
},
promiseTest: () => {
const age = 20;
return new Promise((res, rej) => {
setTimeout(() => res(age), 3000);
// setTimeout(() => rej("error"), 3000);
});
},
callback을 테스트 할 때 fn.callbackTest 까지 실행되면
Jest에서 테스트를 완료해버리므로 3초를 기다리지않고 테스트가 끝나버림.
그래서 done이라는 비어있는 인자를 넘겨주면 Jest가 done이 실행될 때까지 기다려주기 때문에
비동기 테스트를 진행할 수 있음!
test("callback", (done) => {
function callback(name) {
try {
expect(name).toBe("Kim");
done();
} catch (error) {
done(error);
}
}
fn.callbackTest(callback);
});
promise는 return을 써주면 비동기 테스트가 가능함
좀 더 간단하게 resolves / rejects matcher를 사용할 수 있음
// promise
test("promise", () => {
//promise 는 return 이 필요
return fn.promiseTest().then((age) => {
expect(age).toBe(20);
});
//좀더 간단하게(resolves, rejects)
return expect(fn.promiseTest()).resolves.toBe(20);
// return expect(fn.promiseTest()).rejects.toBe("error");
});
async / await 도 테스트 할 수 있음
마찬가지로 좀 더 간단하게 resolves / rejects matcher를 사용할 수 있음
// async await
test("async await", async () => {
// const age = await fn.promiseTest();
// expect(age).toBe(20);
await expect(fn.promiseTest()).resolves.toBe(20);
});
참고
https://inpa.tistory.com/entry/JEST-%F0%9F%93%9A-jest-%EB%AC%B8%EB%B2%95-%EC%A0%95%EB%A6%AC
https://www.youtube.com/watch?v=g4MdUjxA-S4&list=RDCMUCxft4RZ8lrK_BdPNz8NOP7Q&index=4
'Web > JEST' 카테고리의 다른 글
JEST - snapshot (1) | 2023.12.07 |
---|---|
JEST - Mock (2) | 2023.12.06 |
JEST - before, after, only, skip (0) | 2023.12.05 |